We're offering summer camps. Email us to Learn More.
Python
PYTHON
This course ensures students grasp fundamental programming concepts before
applying them to real-world problems, enhancing their learning experience.
​
Week 1: Basic Concepts
Day 1: Introduction to Python Programming
- Overview of Python
- Basic syntax and execution of a Python program
- Writing and executing Python scripts
Day 2: Variables, Data Types, and Basic Operators
- Understanding variables and data types (integers, floats, strings, booleans)
- Arithmetic and assignment operators
- Simple input/output operations
- Practice exercises
Day 3: Control Flow
- Conditional statements (if, elif, else)
- Logical operators
- Introduction to loops (for and while loops)
- Practice exercises
Day 4: Data Structures
- Introducing lists, tuples, and dictionaries
- Basic operations on data structures (creation, modification, access)
- Simple loops and data structures interaction
- Practice exercises
Day 5: Functions and Modules
- Defining and calling functions
- Parameters and return values
- Introduction to Python standard library and modules
- Practice exercises
Week 2: Project-Based Learning
Day 6: Introduction to Project Work
- Overview of project-based learning
- Discussion on project ideas (students can work on individual or group projects based on complexity)
- Planning and outlining the selected project
- Begin project work with instructor support
Day 7: Project Development (Day 1)
- Applying Python basics to project development
- Implementing functions for specific tasks within the project
- Introduction to debugging and troubleshooting code
- Continue project work with instructor guidance
Day 8: Project Development (Day 2)
- Bringing in control flow and data structures to enhance the project
- Efficient data handling and manipulation for project needs
- Continued project development, focusing on achieving project milestones
Day 9: Finalizing Projects
- Implementing any remaining features
- Polishing and refining code, focusing on readability and efficiency
- Testing and debugging to ensure project functionality
- Preparing a simple presentation or documentation of the project
Day 10: Project Presentations and Review
- Students present their projects to the class
- Discussing challenges encountered and how they were overcome
- Review and feedback session with the instructor
- Overview of next steps and further learning resources
This curriculum is designed to offer a balanced mix of theoretical knowledge and practical application, giving students a strong foundation in Python programming. By ending the course with a project, students are able to apply what they've learned in a tangible way, bolstering their confidence and interest in programming.
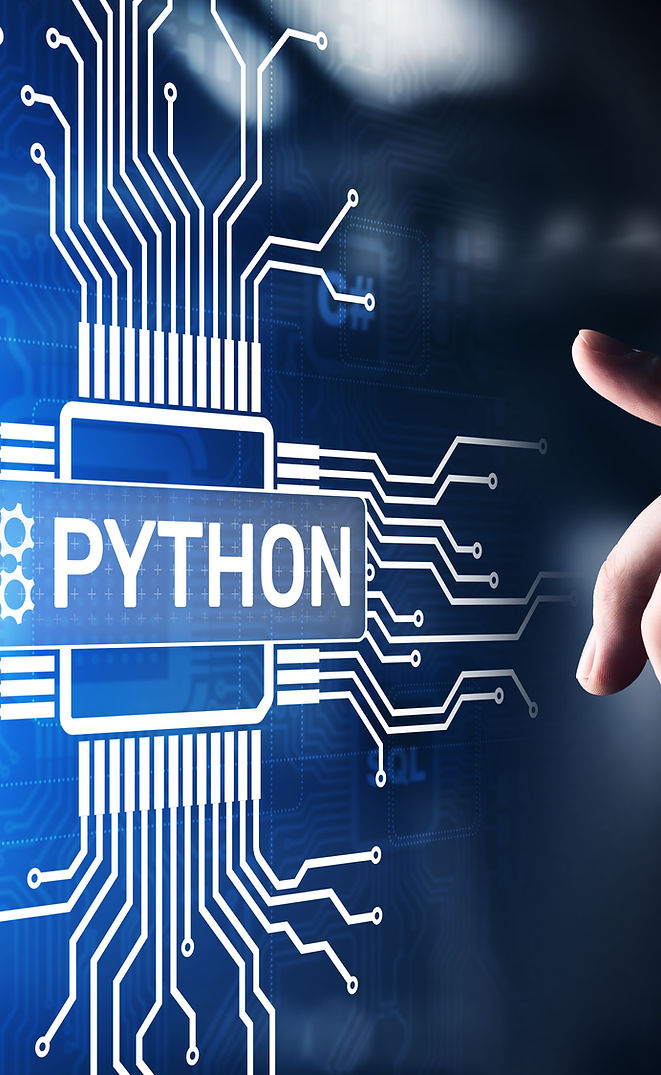
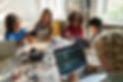
ROBOTICS
The course is structured to introduce students to the fundamentals of robotics and programming, gradually building up to more complex concepts and project-based learning.
Week 1: Introduction to Robotics and Basic Programming
Day 1: Introduction to Robotics
- Overview of robotics and its applications in the real world
- Introduction to the robotics kit and tools that will be used during the course
- Basic safety instructions and teamwork protocols
Day 2: Introduction to Programming
- Introduction to the programming environment
- Basic programming concepts: variables, loops, and conditional statements
- Simple programming exercises related to robotics
Day 3: Sensors and Actuators
- Overview of sensors and actuators and their roles in robotics
- Hands-on activity: experimenting with different types of sensors (e.g., ultrasonic, touch, light) and actuators (e.g., motors)
- Programming robots to respond to sensor inputs
Day 4: Introduction to Robot Control
- Basics of robot movement and control (e.g., moving forward, turning)
- Programming exercises focused on controlling the robot's movements
- Introduction to debugging techniques for robotics programming
Day 5: Project Day 1
- Application of learned concepts in a mini-project
- Teams plan their project, outline the necessary steps, and start programming
Week 2: Advanced Robotics Concepts and Projects
Day 6: Advanced Sensors and Data Handling
- Delve deeper into advanced sensor usage and managing sensor data
- Introduction to data structures useful in robotics projects
Day 7: Introduction to Autonomous Behaviors
- Concepts of autonomy in robotics and examples of autonomous robots
- Programming robots to perform tasks autonomously
- Exercises to practice autonomous control and behavior programming
Day 8: Project Planning and Troubleshooting
- Students brainstorm and finalize their project ideas for the end-of-course project
- Discussion on project planning, division of labor, and troubleshooting strategies for common issues
Day 9: Project Development Day
- Students work in teams on their projects, applying the concepts learned throughout the course.
- Instructors provide support, guidance, and troubleshooting assistance as needed.
Day 10: Project Presentations and Course Wrap-Up
- Teams present their projects to the class, explaining their design choices, challenges faced, and how they overcame them
- Reflection on the learning process and discussion on how to further explore robotics and programming
- Instructor feedback session
Each day includes a balance of theoretical instruction, hands-on activities, and reflection/discussion to consolidate learning.

PYGAME
The course focuses on hands-on projects that reinforce learning and spark creativity.
​
Week 1: Introduction to Pygame and Basic Concepts
Day 1: Getting Started with Python and Pygame
- Introduction to Python programming
- Setting up Pygame and creating a basic window
- Drawing shapes and handling window events
Day 2: Graphics and Animation
- Loading and displaying images
- Animating sprites
- Understanding game loops
Day 3: Game Mechanics
- User input and event handling
- Movement and physics basics
- Collision detection fundamentals
Day 4: Designing a Simple Game
- Planning a game project
- Defining game objects and their interactions
- Sketching game flow and designing levels
Day 5: Starting the Game Project
- Implementing the game loop
- Adding player-controlled characters
- Creating a simple level
Week 2: Advanced Topics and Project Work
Day 6: Advanced Game Mechanics
- Implementing score and health systems
- Enhancing game physics
- Adding sound effects and background music
Day 7: More on Graphics and Animation
- Advanced animation techniques
- Using tilemaps for game levels
Day 8: Game Polish
- Menu systems and game states (start, game over, etc.)
- Adding special effects
- UI elements
Day 9 & 10: Final Project and Presentation
- Students use these days to complete their game projects, implementing all the concepts learned
- Adding final touches and polishing the game
- Preparation for project presentations
**Final Presentation:**
Students showcase their projects to the class. This involves demonstrating their game, explaining their code, and discussing challenges they overcame.
This curriculum aims to not only teach the technical skills required for game development but also to foster creativity, problem-solving, and the joy of bringing one's imagination to life through programming.

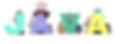
JAVA
This course is designed to introduce beginners to the fundamentals of Java programming. The first week focuses on basic concepts and syntax, while the second week is dedicated to applying these concepts in a project-based approach.
Week 1: Understanding Java Basics
Day 1: Introduction to Java
- Overview of Java (History, Versions, Features)
- Understanding Java Syntax and Basic Program Structure
- Writing Your First Java Program: The “Hello World”
Day 2: Basic Concepts in Java
- DataTypes and Variables
- Operators and Expressions
- Input/Output Operations in Java
- Practical Exercises
Day 3: Control Flow Statements
- Conditional Statements (if, if-else, switch)
- Looping Statements (for, while, do-while)
- Practice Problems to Reinforce Learning
Day 4: Working with Arrays and Methods
- Introduction to Arrays and Its Usage
- Single-Dimensional and Multi-Dimensional Arrays
- Introduction to Methods in Java
- Method Overloading
- Exercises on Arrays and Methods
Day 5: OOP Basics in Java
- Introduction to Object-Oriented Programming (OOP)
- Classes and Objects
- Basic Principles of OOP (Encapsulation, Inheritance)
- Creating Basic Classes and Objects in Java
Week 2: Project-Based Learning and Advanced Topics
Day 6: Advanced OOP Concepts
- Deep Dive into Inheritance
- Introduction to Polymorphism
- Abstract Classes and Interfaces
- Practical Implementation in Java
Day 7: Exception Handling and File I/O
- Understanding Exception Handling
- Try-Catch Block and Multiple Catch Blocks
- Understanding File I/O in Java
- Reading from and Writing to Files
Day 8: Introduction to GUI Programming
- Basics of Java Swing
- Creating Simple User Interfaces with Swing
- Event Handling
Day 9: Planning and Designing the Project
- Brainstorming Session to Decide the Project Theme
- Introduction to Project Requirements and Planning
- Start Coding with Guidance from Instructors
Day 10: Project Development and Presentation
- Continued Development with Instructor Support
- Final Touches and Debugging
- Presentation of Projects to the Class
- Feedback Session